LangSmith SDK for .NET

Features 🔥
- Fully generated C# SDK based on official OpenAPI specification using AutoSDK
- Automatic releases of new preview versions if there was an update to the OpenAPI specification
- All modern .NET features - nullability, trimming, NativeAOT, etc.
- Support .Net Framework/.Net Standard 2.0
Usage
Initializing
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60 | using var client = new LangSmithClient();
using var openAiClient = new OpenAiClient();
client.JsonSerializerContext = new SpecialJsonSerializerContext(tryAGI.OpenAI.SourceGenerationContext.Default);
// This can be a user input to your app
var question = "Can you summarize this morning's meetings?";
// This can be retrieved in a retrieval step
const string context = "During this morning's meeting, we solved all world conflict.";
var messages = new[]
{
"You are a helpful assistant. Please respond to the user's request only based on the given context."
.AsSystemMessage(),
$"Question: {question}\\nContext: {context}",
};
// Create parent run
var parentRunId = Guid.NewGuid();
await client.Run.CreateRunAsync(
name: "Chat Pipeline",
runType: CreateRunRequestRunType.Chain,
id: parentRunId,
inputs: new Dictionary<string, object>
{
["question"] = question,
});
// Create child run
var childRunId = Guid.NewGuid();
await client.Run.CreateRunAsync(
name: "OpenAI Call",
runType: CreateRunRequestRunType.Llm,
id: childRunId,
parentRunId: parentRunId,
inputs: new Dictionary<string, object>
{
["messages"] = messages,
});
// Generate a completion
var chatCompletion = await openAiClient.Chat.CreateChatCompletionAsync(
model: CreateChatCompletionRequestModel.Gpt35Turbo,
messages: messages);
// End runs
await client.Run.UpdateRunAsync(
runId: childRunId,
outputs: new Dictionary<string, object>
{
["chatCompletion"] = chatCompletion,
},
endTime: DateTime.UtcNow.ToString("O"));
await client.Run.UpdateRunAsync(
runId: parentRunId,
outputs: new Dictionary<string, object>
{
["answer"] = chatCompletion.Choices[0].Message.Content ?? string.Empty,
},
endTime: DateTime.UtcNow.ToString("O"));
|
Support
Priority place for bugs: https://github.com/tryAGI/LangSmith/issues
Priority place for ideas and general questions: https://github.com/tryAGI/LangSmith/discussions
Discord: https://discord.gg/Ca2xhfBf3v
Acknowledgments
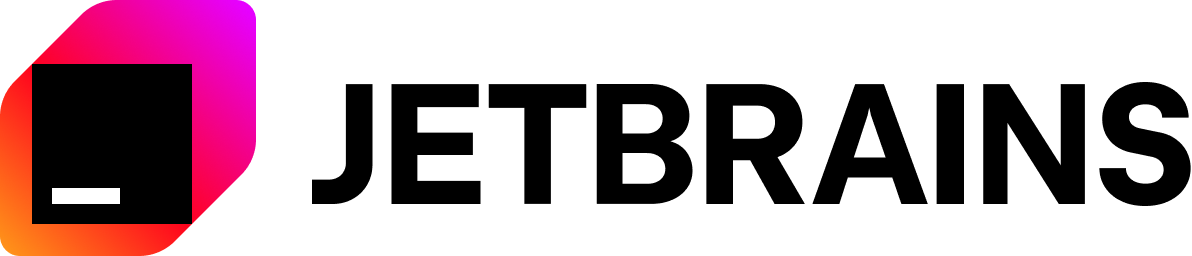
This project is supported by JetBrains through the Open Source Support Program.

This project is supported by CodeRabbit through the Open Source Support Program.